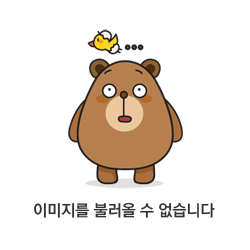
✔️ JdbcTemplate
SQL 직접 사용시 JDBCTemplate는 매우 좋다.
spring-jdbc 라이브러리에 포함, 별도의 복잡한 설정 없이 바로 사용 가능
반복 문제 해결
- 템플릿 콜백 패턴 사용
- 개발자는 sql 작성, 전달 파라미터 정의, 응답 값 매핑만 하면 된다.
반복 문제?
커넥션 획득
statement 를 준비하고 실행
결과를 반복하도록 루프 실행
커넥션 종료, statement, resultset 종료
트랜잭션 다루기 위한 커넥션 동기화
예외 발생시 스프링 예외 변환기 실행
단점 : 동적 SQL 해결 어려움
종류
JdbcTemplate : 기본이며 순서대로 바인딩 (where name = ?)
NamedParameterJdbcTemplate : 이름 지정 바인 (where name = :username)
SimpleJdbcInsert : datasource로 생성, Insert SQL를 직접 작성하지 않아도 편리하게 기능 제공
제공 메서드
query(sql, param, RowMapper) return T or List<T> // 범용
queryForObject(sql, param, Class<T> requiredType) return T // 단건 조회
queryForList(sql, param) return List<Map<String, Object> // 여러개 조회
//insert, update, delete
update(sql, param) return int // 변경된 행 개수 반환
execute() // DDL 등에 사용 가능
bactchUpdate() // bulk insert용
template.query(sql, param, rowMapper)
파라미터(param)
sql에 파라미터 바인딩
- Map
- MapSqlParameterSource
- addValue(key , value)
- addValues(Map<String, ?>) // Map 형태로 한번에 여러 파라미터를 넘김
- BeanPropertySqlParameterSource(Object o) // 빈 프로퍼티 규약에 따라 자동으로 파라미터 객체 생성
- getName() -> name // 카멜 표기법으로 나타냄
- key = name , value = 이름명 값
RowMapper
ResultSet의 row를 매핑하는 JdbcTemplate 인터페이스
즉, ResultSet을 하나씩 읽어와 객체에 매핑해준다.
- 람다식 (rs, rowNum) -> new 객체()
- RowMapper<T extends Object>
- BeanPropertyMapper.newInstance(class) // ResultSet의 결과를 받아서 자바빈 규악에 맞추어 데이터를 변환
@Slf4j
@Repository
public class JdbcRepository {
private final NamedParameterJdbcTemplate template;
private final SimpleJdbcInsert jdbcInsert; // insert sql을 편리하게 사용
// datasource로 생성해야함.
public JdbcRepository(DataSource dataSource) {
this.template = new NamedParameterJdbcTemplate(dataSource);
this.jdbcInsert = new SimpleJdbcInsert(dataSource)
.withTableName("users")// 데이터를 저장할 테이블 명 지정
.usingGeneratedKeyColumns("id"); // key를 생성하는 PK 컬럼 명 지정
// .usingColumns(); 특정 값만 저장하고 싶을때 컬럼 지정
}
// jdbc에서 파라미터 넘기는방법
public Optional<User> paramMapper() {
String sql = "select * from users where username =:username";
Map<String, String> map = Map.of("username", "hh");// 1번 Map으로 전달
SqlParameterSource source = new MapSqlParameterSource()
.addValue("username", "hh"); // 2번 MapSqlParameterSource 객체로 전달
template.query(sql, map, userRowMapper());
template.query(sql, source, userRowMapper());
template.query(sql, source, (rs, rowNum) -> new User(rs.getString("username"), rs.getString("password")));
template.query(sql, source, userRowMapper());
// insert할때만 사용
Number idKey = jdbcInsert.executeAndReturnKey(source); // 실행하고 키 반환
// bean으로 객체를 넣어서 바로 전달
BeanPropertySqlParameterSource parameterSource = new BeanPropertySqlParameterSource(new User("hh", "psw"));
jdbcInsert.executeAndReturnKey(parameterSource);
idKey.intValue();
return null;
}
private RowMapper<User> userRowMapper() {
return BeanPropertyRowMapper.newInstance(User.class);
}
}
🔖 학습내용 출처
'Back-End > Spring' 카테고리의 다른 글
filter 코드 리팩토링 해보기 (0) | 2025.04.07 |
---|---|
JWT(Json Web Token) (0) | 2025.03.15 |